[ad_1]
On this instructional, you’ll find out how we at ARKx crafted the audio-reactive visuals for Coala Tune’s web site. We’ll stroll throughout the ideas and strategies used to synchronize audio frequencies and pace, making a dynamic visualizer with procedural particle animations.
Getting Began
We can initialize our 3.js scene handiest after the consumer interacts; this manner, we will be able to allow the audio to autoplay and steer clear of the block coverage of the primary browsers.
export default magnificence App {
constructor() {
this.onClickBinder = () => this.init()
file.addEventListener('click on', this.onClickBinder)
}
init() {
file.removeEventListener('click on', this.onClickBinder)
//BASIC THREEJS SCENE
this.renderer = new THREE.WebGLRenderer()
this.digicam = new THREE.PerspectiveCamera(70, window.innerWidth / window.innerHeight, 0.1, 10000)
this.scene = new THREE.Scene()
}
}
Examining Audio Knowledge
Subsequent, we initialize our Audio and BPM Managers. They’re accountable for loading the audio, inspecting it, and synchronizing it with the visible components.
async createManagers() {
App.audioManager = new AudioManager()
look forward to App.audioManager.loadAudioBuffer()
App.bpmManager = new BPMManager()
App.bpmManager.addEventListener('beat', () => {
this.debris.onBPMBeat()
})
look forward to App.bpmManager.detectBPM(App.audioManager.audio.buffer)
}
The AudioManager
magnificence then a lot the audio from a URL—we’re the usage of a Spotify Preview URL—and analyzes it to wreck down the audio alerts into frequency packing containers in real-time.
const audioLoader = new THREE.AudioLoader();
audioLoader.load(this.music.url, buffer => {
this.audio.setBuffer(buffer);
})
Frequency Knowledge
We need to segregate the frequency spectrum into low, mid, and top bands to calculate the amplitudes.
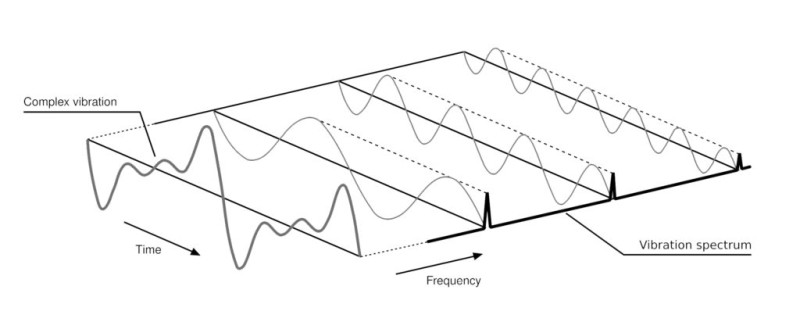
To phase the bands, we wish to outline get started and finish issues (e.g., the low band vary begins on the lowFrequency
worth and ends on the midFrequency
get started worth). To get the typical amplitude, merely multiply the frequencies via the buffer duration, then divide via the pattern fee, and normalize it to a 0-1 scale.
this.lowFrequency = 10;
this.frequencyArray = this.audioAnalyser.getFrequencyData();
const lowFreqRangeStart = Math.flooring((this.lowFrequency * this.bufferLength) / this.audioContext.sampleRate)
const lowFreqRangeEnd = Math.flooring((this.midFrequency * this.bufferLength) / this.audioContext.sampleRate)
const lowAvg = this.normalizeValue(this.calculateAverage(this.frequencyArray, lowFreqRangeStart, lowFreqRangeEnd));
//THE SAME FOR MID AND HIGH
Detecting Pace
The amplitude of the frequencies isn’t sufficient to align the song beat with the visible components. Detecting the BPM (Beats In line with Minute) is very important to make the weather react in sync with the heartbeat of the song. In Coala’s undertaking, we supply many songs from their artists’ label, and we don’t know the pace of every piece of song. Subsequently, we’re detecting the BPM asynchronously the usage of the fantastic web-audio-beat-detector
module, via merely passing the audioBuffer.
const { bpm } = look forward to wager(audioBuffer);
Dispatching the Alerts
After detecting the BPM, we will be able to dispatch the development sign the usage of setInterval
.
this.period = 60000 / bpm; // Convert BPM to period
this.intervalId = setInterval(() => {
this.dispatchEvent({ sort: 'beat' })
}, this.period);
Procedural Reactive Debris (The joys section 😎)
Now, we’re going to create our dynamic debris that may quickly be attentive to audio alerts. Let’s get started with two new purposes that may create elementary geometries (Field
and Cylinder
) with random segments and homes; this method will lead to a novel construction every time.
Subsequent, we’ll upload this geometry to a THREE.Issues
object with a easy ShaderMaterial
.
const geometry = new THREE.BoxGeometry(1, 1, 1, widthSeg, heightSeg, depthSeg)
const subject material = new THREE.ShaderMaterial({
aspect: THREE.DoubleSide,
vertexShader: vertex,
fragmentShader: fragment,
clear: true,
uniforms: {
measurement: { worth: 2 },
},
})
const pointsMesh = new THREE.Issues(geometry, subject material)
Now, we will be able to start growing our meshes with random attributes in a specified period:
Including noise
We drew inspiration from Akella’s FBO Educational and integrated the curl noise into the vertex shader to create natural, natural-looking actions and upload fluid, swirling motions to the debris. I received’t delve deeply into the reason of Curl Noise and FBO Debris, as Akella did an incredible process in his instructional. You’ll be able to test it out to be informed extra about it.
Animating the debris
To summarize, within the vertex shader, we animate the issues to succeed in dynamic results that dictate the debris’ conduct and look. Beginning with newpos
, which is the unique place of every level, we create a goal. This goal provides curl noise alongside its customary vector, various in response to frequency and amplitude uniforms. It’s interpolated via the ability of the gap d
between them. This procedure creates a easy transition, easing out as the purpose approaches the objective.
vec3 newpos = place;
vec3 goal = place + (customary * .1) + curl(newpos.x * frequency, newpos.y * frequency, newpos.z * frequency) * amplitude;
waft d = duration(newpos - goal) / maxDistance;
newpos = combine(place, goal, pow(d, 4.));
We additionally upload a wave movement to newpos.z
, including an additional layer of liveliness to the animation.
newpos.z += sin(time) * (.1 * offsetGain);
Additionally, the dimensions of every level adjusts dynamically in response to how shut the purpose is to its goal and its intensity within the scene, making the animation really feel extra third-dimensional.
gl_PointSize = measurement + (pow(d,3.) * offsetSize) * (1./-mvPosition.z);
Right here it’s:
Including Colours
Within the fragment shader, we’re protecting out the purpose with a circle form serve as and interpolating the startColor
and endColor
uniforms in step with the purpose’s vDistance
outlined within the vertex:
vec3 circ = vec3(circle(uv,1.));
vec3 colour = combine(startColor,endColor,vDistance);
gl_FragColor=vec4(colour,circ.r * vDistance);
Bringing Audio and Visuals In combination
Now, we will be able to use our creativity to assign the audio knowledge and beat to all of the homes, each within the vertex and fragment shader uniforms. We will additionally upload some random animations to the size, place and rotation the usage of GSAP.
replace() {
// Dynamically replace amplitude in response to the top frequency knowledge from the audio supervisor
this.subject material.uniforms.amplitude.worth = 0.8 + THREE.MathUtils.mapLinear(App.audioManager.frequencyData.top, 0, 0.6, -0.1, 0.2)
// Replace offset achieve in response to the low frequency knowledge for refined impact adjustments
this.subject material.uniforms.offsetGain.worth = App.audioManager.frequencyData.mid * 0.6
// Map low frequency knowledge to a spread and use it to increment the time uniform
const t = THREE.MathUtils.mapLinear(App.audioManager.frequencyData.low, 0.6, 1, 0.2, 0.5)
this.time += THREE.MathUtils.clamp(t, 0.2, 0.5) // Clamp the worth to verify it remains inside a desired vary
this.subject material.uniforms.time.worth = this.time
}
Conclusion
This instructional has guided you on the best way to synchronize sound with attractive visible particle results the usage of 3.js.
Hope you loved it! If in case you have questions, let me know on Twitter.
Credit
[ad_2]