[ad_1]
I’ve numerous bother operating with reactive streams. It is simply no longer how my mind works absolute best. Give me a easy event-stream, and I will most commonly dangle that during my head. However, begin to mix streams in combination, and my mind freezes up like a deer in headlights – my eyes darting from operator to operator, desperately seeking to build-up a psychological type of what’s in truth taking place. As such, I lately got rid of RxJS from an software and changed the reactive streams with state machines. It is no doubt extra code; however, I to find it more uncomplicated to reason why about and deal with.
Run this demo in my JavaScript Demos challenge on GitHub.
View this code in my JavaScript Demos challenge on GitHub.
ASIDE: My need to interchange RxJS on this specific context was once pushed essentially via the sheer quantity of “seller” code that was once being pulled-in. That is an outdated app and not using a tree-shaking and it was once pulling in actually hundreds-of-kilobytes of RxJS and most effective being utilized in two puts. My primary goal was once in decreasing the seller payload. Making the code more uncomplicated to reason why about (for me individually) was once a side-effect.
I would possibly not reproduce the whole thing of the RxJS code right here, however I’m going to come up with a high-level sense of the way the streams have been being blended. The context for this code is a mouse-drag observer (following via some window-scrolling, no longer proven). Expectantly I did not spoil the code right here an excessive amount of via seeking to pare it down.
// Get the 3 main occasions
var mouseup = Rx.Observable.fromEvent(record, 'mouseup');
var mousemove = Rx.Observable.fromEvent(record, 'mousemove');
var mousedown = Rx.Observable.fromEvent(dragTarget, 'mousedown');
var intervalSource = Rx.Observable.period(60, Rx.Scheduler.requestAnimationFrame);
var mousedrag = mousedown.flatMap(serve as(md) {
go back intervalSource
.takeUntil(mouseup)
.withLatestFrom(mousemove, serve as(s1, s2) {
go back s2.clientY;
})
.scan(md.clientY, serve as( initialClientY, clientY ) {
go back( clientY - initialClientY );
})
;
});
this.subscription = mousedrag.subscribe(serve as( delta ) {
console.log( "Drag delta from foundation:", delta );
});
I am certain – or, slightly, I suppose – that for those who have been talented in RxJS, this code would make sense at a look. However, I am not very acquainted with the RxJS operator APIs (which is section of why I to find RxJS so complicated). So, once I have a look at this code, it isn’t straight away glaring the way it all suits in combination. Obviously, it has one thing to do with mouse actions; however, if I needed to describe the total intent of the code, it might take me some time to build a significant psychological type.
After including numerous console.log()
statements to those circulate callback, I used to be in a position to piece in combination the overall regulate glide:
- Person mouses-down.
- We begin an period circulate that emits an occasion ever 60ms.
- We learn from the period circulate till the consumer mouses-up.
- When the consumer strikes their mouse, we emit an occasion with the present mouse place.
- We mix the period occasion with the mouse motion occasion and calculate the gap the mouse has moved relative to the unique mouse-down occasion.
With a view to translate this RxJS into code that I may perceive and deal with extra simply, I began to consider the states that those streams constitute. I got here up with:
-
Default State: The consumer is solely sitting there staring on the display screen.
-
Pending State: The consumer has moused-down, however hasn’t moved their mouse but. At this level, we do not know if they’re “clicking”; or, if they’re about to begin “dragging”.
-
Dragging State: The consumer is transferring their mouse whilst the button continues to be pressed.
None of those states exists in isolation. The entire interplay works via transitioning from one state to any other in line with some kind of occasion:
- Default →
mousedown
→ Pending - Pending →
mouseup
→ Default - Pending →
mousemove
(past threshold) → Dragging - Dragging →
mouseup
→ Default
There are all method of libraries available in the market for outlining and eating state machines. However, for this exploration, I’ll stay it easy. Every state is outlined via a sequence of Purposes whose names are prefixed with the present state. As an example, default_setup()
, is the initialization means for the “Default” state.
Every state has each a setup
and a teardown
means that is known as when getting into and exiting a given state, respectively. So, the Default state has each a default_setup()
and a default_teardown()
means. Every state defines its personal event-handlers, which is how one state is aware of when and why to transition to the following state.
The next code is my try to recreate the RxJS occasion streams with this 3-state machine:
NOTE: In my method, the “pending” state has to go a given drag-threshold earlier than transferring into the “dragging” state. This constraint was once no longer a part of the RxJS occasion streams; however, I feel it must were. That mentioned, it isn’t glaring to me how I might have added it to the given RxJS code.
<!doctype html>
<html lang="en">
<frame>
<h1>
Changing RxJS With A State System In JavaScript
</h1>
<p>
In the event you mouse-down after which get started dragging (vertically), you get console logging.
</p>
<script kind="textual content/javascript">
default_setup();
// ---
// DEFAULT STATE: At this level, the consumer is solely viewing the web page, however has no longer
// but interacted with it. After they mousedown, we're going to transfer into the pending state.
// ---
serve as default_setup() {
console.information( "Default: Setup" );
record.addEventListener( "mousedown", default_handleMousedown );
}
serve as default_teardown() {
console.information( "Default: Teardown" );
record.removeEventListener( "mousedown", default_handleMousedown );
}
serve as default_handleMousedown( occasion ) {
occasion.preventDefault();
default_teardown();
pending_setup( occasion.clientY );
}
// ---
// PENDING STATE: The consumer has moused-down at the web page, however we do not but know if
// they intend to tug or simply click on. In the event that they do begin to drag (and go a minimal
// threshold), we're going to transfer into the dragging state.
// ---
serve as pending_setup( clientY ) {
console.information( "Pending: Setup" );
record.addEventListener( "mouseup", pending_handleMouseup );
record.addEventListener( "mousemove", pending_handleMousemove );
pending_setup.initialClientY = clientY;
}
serve as pending_teardown() {
console.information( "Pending: Teardown" );
record.removeEventListener( "mouseup", pending_handleMouseup );
record.removeEventListener( "mousemove", pending_handleMousemove );
}
serve as pending_handleMouseup( occasion ) {
pending_teardown();
default_setup();
}
serve as pending_handleMousemove( occasion ) {
// Handiest transfer onto subsequent state if dragging threshold is handed.
// --
// CAUTION: This idea was once no longer provide within the RxJS model; however, I feel it
// must were. And, within the state-based method (for me) it's more uncomplicated to
// reason why about this replace the usage of states vs. streams.
if ( Math.abs( pending_setup.initialClientY - occasion.clientY ) > 10 ) {
pending_teardown();
dragging_setup( pending_setup.initialClientY );
}
}
// ---
// DRAGGING STATE.
// ---
serve as dragging_setup( clientY ) {
console.information( "Dragging: Setup" );
record.addEventListener( "mousemove", dragging_handleMousemove );
record.addEventListener( "mouseup", dragging_handleMouseup );
dragging_setup.initialClientY = clientY;
dragging_setup.currentClientY = null;
dragging_setup.timer = setInterval( dragging_handleInterval, 60 );
}
serve as dragging_teardown() {
console.information( "Dragging: Teardown" );
record.removeEventListener( "mousemove", dragging_handleMousemove );
record.removeEventListener( "mouseup", dragging_handleMouseup );
clearInterval( dragging_setup.timer );
}
serve as dragging_handleMousemove( occasion ) {
dragging_setup.currentClientY = occasion.clientY;
}
serve as dragging_handleMouseup( occasion ) {
dragging_teardown();
default_setup();
}
serve as dragging_handleInterval() {
console.log(
"Drag delta from foundation:",
( dragging_setup.currentClientY - dragging_setup.initialClientY )
);
}
</script>
</frame>
</html>
So, we’ve got long past from 21-lines of RxJS occasion streams as much as 123-lines of State Machines. That is nearly 6x the quantity of code. It would look like we are transferring within the mistaken path. However – for me individually – once I have a look at this code, it is a lot more uncomplicated to know. Moreover, I do not also have to completely perceive the entire code at one time with the intention to consider successfully; all I’ve to do is have a look at the present state and consider the way it transitions to the subsequent state.
If we run this code within the browser and drag the mouse round, we get the next output:
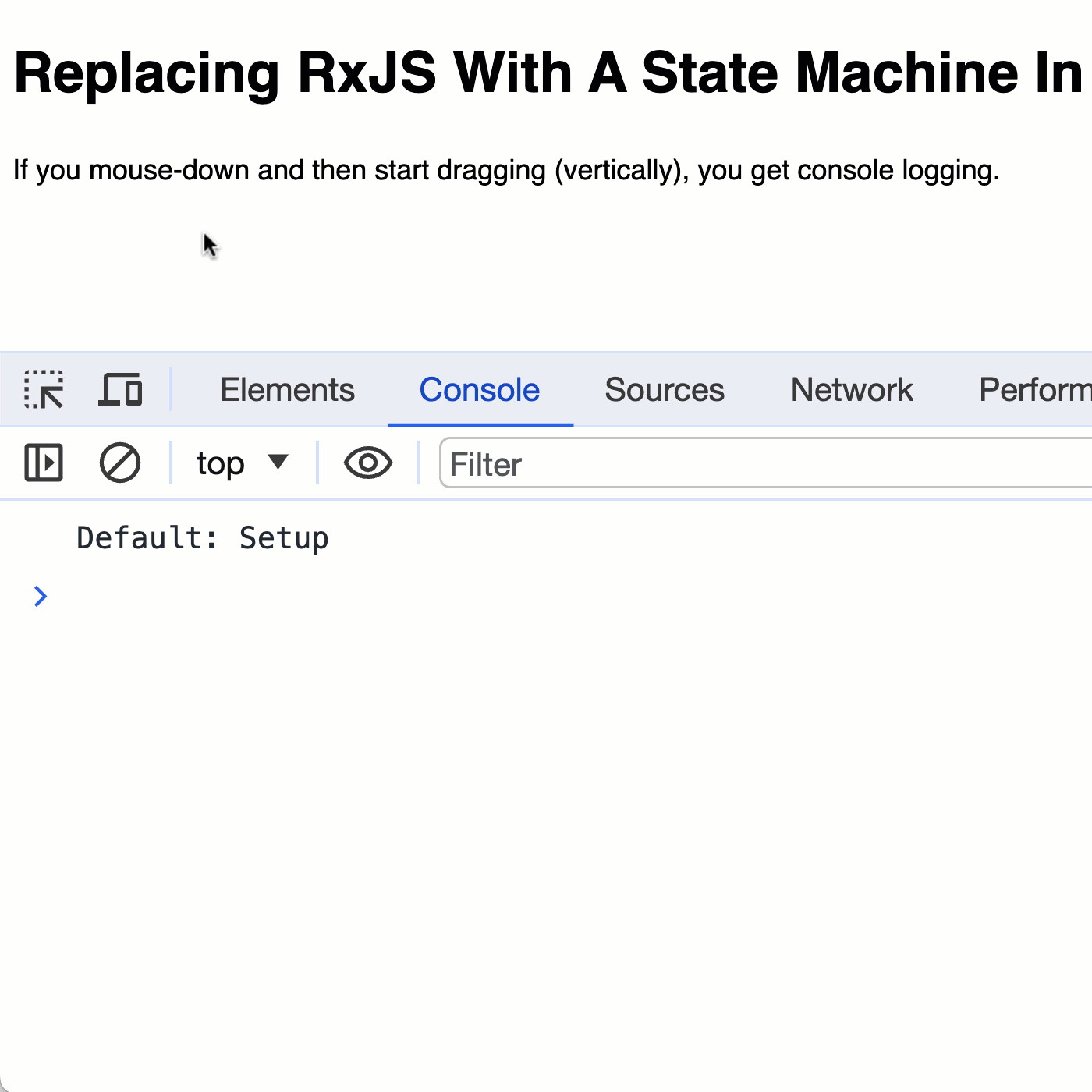
As you’ll see, we are logging the setup and teardown occasions for each and every state. And, we are logging-out the delta calculated throughout the dragging state. This state gadget code works as meant.
That is no longer an issue in opposition to RxJS. I do know that many of us completely love RxJS and really feel that it very much simplifies the code that they write. I am simply no longer a kind of other people. Reactive occasion streams overload my mind. I to find it a lot more uncomplicated to assume with regards to “states”; and, I do not thoughts that it takes extra code to make that occur.
Epilogue on Unsubscribing
Within the RxJS model of the code, a subscription
object is created. When the present software View is “destroyed”, that subscription object can also be “disposed”, which can, in flip, mechanically forestall all of the upstream occasions from firing. That is lovely great!
In my state gadget method, when the present software View is “destroyed”, I might merely name all 3 of the *_teardown()
strategies (since I may no longer know which state is these days lively). That is quite much less sublime; however, once more, I am OK with that.
Wish to use code from this put up?
Take a look at the license.
[ad_2]