[ad_1]
Sorting algorithms are a elementary a part of laptop science and feature numerous packages, starting from sorting information in databases to organizing track playlists. However what precisely are sorting algorithms, and the way do they paintings? We’ll solution that query on this article through offering a complete take a look at the differing types algorithms and their makes use of, together with pattern code.
We may get a little technical right here and there, like the usage of giant O notation to investigate time complexity and house complexity of various algorithms. However we’ll additionally supply high-level overviews that are meant to simply be understood through maximum.
It’s an extended learn evidently, so let’s get to it!
Contents:
- What Is a Sorting Set of rules?
- What Are Sorting Algorithms Used For?
- Why Are Sorting Algorithms So Necessary?
- The Other Sorts of Sorting in Knowledge Constructions
- Best 10 Sorting Algorithms You Want to Know
- All Sorting Algorithms When put next
- What’s the Maximum Commonplace Sorting Set of rules?
What Is a Sorting Set of rules?
Necessarily, a sorting set of rules is a pc program that organizes information into a particular order, corresponding to alphabetical order or numerical order, normally both ascending or descending.
What Are Sorting Algorithms Used For?
Sorting algorithms are principally used to arrange massive quantities of information in an effective approach in order that it may be searched and manipulated extra simply. They’re extensively utilized to enhance the potency of different algorithms corresponding to looking out and merging, which depend on taken care of information for his or her operations.
Why Are Sorting Algorithms So Necessary?
Sorting algorithms are used to arrange information in a specific order, which makes it more uncomplicated to look, get right of entry to, and analyze. In lots of packages, sorting is a essential a part of the knowledge processing pipeline, and the potency of the sorting set of rules could have an important affect at the general efficiency of the machine.
-
In databases. Sorting is used to retrieve data in a specific order, corresponding to through date, alphabetical order, or numerical order. This permits customers to temporarily to find the knowledge they want, with no need to manually seek thru massive quantities of unsorted information.
-
In search engines like google and yahoo. To rank seek ends up in order of relevance. By means of sorting the ends up in this manner, customers can temporarily to find the ideas they’re on the lookout for, with no need to sift thru beside the point or unrelated effects.
-
In lots of medical and engineering packages. Researchers can run information research and simulations to achieve insights into complicated methods and make extra correct predictions about long term habits.
The Other Sorts of Sorting in Knowledge Constructions
There are more than a few varieties of sorting to be had. The number of sorting set of rules is dependent upon more than a few components, corresponding to the scale of the knowledge set, the kind of information being taken care of, and the specified time and house complexity.
Comparability-based sorting algorithms
Those examine parts of the knowledge set and resolve their order in line with the results of the comparability. Examples of comparison-based sorting algorithms come with bubble kind, insertion kind, quicksort, merge kind, and heap kind.
Non-comparison-based sorting algorithms
Those don’t examine parts without delay, however moderately use different houses of the knowledge set to resolve their order. Examples of non-comparison-based sorting algorithms come with counting kind, radix kind, and bucket kind.
In-place sorting algorithms
Those algorithms kind the knowledge set in-place, which means they don’t require further reminiscence to retailer intermediate effects. Examples of in-place sorting algorithms come with bubble kind, insertion kind, quicksort, and shell kind.
Solid sorting algorithms
Those keep the relative order of equivalent parts within the information set. Examples of strong sorting algorithms come with insertion kind, merge kind, and Timsort.
Adaptive sorting algorithms
Those profit from any present order within the information set to enhance their potency. Examples of adaptive sorting algorithms come with insertion kind, bubble kind, and Timsort.
Best 10 Sorting Algorithms You Want to Know
Let’s now undergo ten of the highest sorting algorithms to pay attention to when having a look to select one.
Bubble kind
Bubble kind is a straightforward sorting set of rules that many times steps thru a given listing of things, evaluating every pair of adjoining pieces and swapping them in the event that they’re within the flawed order. The set of rules continues till it makes a cross thru all of the listing with out swapping any pieces.
Bubble kind could also be every so often known as “sinking kind”.
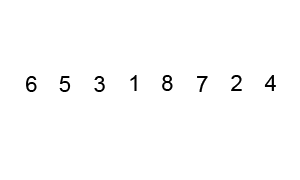
The historical past of bubble kind
The origins of bubble kind hint again to the past due Fifties, with Donald Knuth popularizing it in his vintage 1968 e-book The Artwork of Pc Programming.
Since then, it’s been extensively utilized in more than a few packages, together with sorting algorithms for compilers, sorting parts in databases, or even within the sorting of enjoying playing cards.
Benefits and downsides of bubble kind
Bubble kind is thought of as to be a somewhat inefficient sorting set of rules, as its common and worst-case complexity are each $O(n^2)$
. This makes it a lot much less environment friendly than maximum different sorting algorithms, corresponding to quicksort or mergesort.
Technical Observe: $O(n^2)$
complexity implies that the time it takes for an set of rules to complete is proportional to the sq. of the scale of the enter. Which means that greater enter sizes purpose the set of rules to take considerably longer to finish.
For instance, if you happen to believe an set of rules that types an array of numbers, it will take one 2d to kind an array of ten numbers, however it would take 4 seconds to kind an array of 20 numbers. It is because the set of rules will have to examine every part within the array with each and every different part, so it will have to do 20 comparisons for the bigger array, in comparison to simply ten for the smaller array.
It’s, then again, quite simple to grasp and enforce, and it’s continuously used as an creation to kind and as a development block for extra complicated algorithms. However at the present time it’s infrequently utilized in follow.
Use instances for bubble kind
Bubble kind is a straightforward set of rules that can be utilized for sorting small lists or arrays of parts. It’s simple to enforce and perceive, and subsequently can be utilized in scenarios the place simplicity and readability are extra vital than efficiency.
-
Tutorial functions. It’s continuously utilized in laptop science lessons for instance of a easy sorting set of rules. Scholars can know about elementary sorting ways and acquire an figuring out of ways algorithms paintings through finding out bubble kind.
-
Sorting small information units. It may be used for sorting small information units of as much as a couple of hundred parts. In instances the place efficiency isn’t a essential worry, bubble kind is usually a fast and simple option to kind small lists.
-
Pre-sorting information. It may be used as a initial step in additional complicated sorting algorithms. For instance, if the knowledge is already partly taken care of, bubble kind can be utilized to additional kind the knowledge prior to working a extra complicated set of rules.
-
Sorting information with restricted assets. It’s helpful in scenarios the place assets are restricted, corresponding to in embedded methods or microcontrollers, as it calls for little or no reminiscence and processing energy.
-
Development blocks for extra complicated algorithms. It’s continuously used along with merge kind or quicksort, and sorting small subarrays with insertion kind, given those different algorithms can succeed in higher efficiency on greater information units.
Bubble kind implementation
- Use nested loops to iterate thru pieces.
- Examine adjoining pieces within the listing.
- Change pieces if they’re within the flawed order.
- Proceed till the listing is taken care of.
Bubble kind in Python
def bubble_sort(pieces):
for i in fluctuate(len(pieces)):
for j in fluctuate(len(pieces)-1-i):
if pieces[j] > pieces[j+1]:
pieces[j], pieces[j+1] = pieces[j+1], pieces[j]
go back pieces
pieces = [6,20,8,19,56,23,87,41,49,53]
print(bubble_sort(pieces))
Bubble kind in JavaScript
serve as bubbleSort(pieces) {
let swapped;
do {
swapped = false;
for (let i = 0; i < pieces.size - 1; i++) {
if (pieces[i] > pieces[i + 1]) {
let temp = pieces[i];
pieces[i] = pieces[i + 1];
pieces[i + 1] = temp;
swapped = true;
}
}
} whilst (swapped);
go back pieces;
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(bubbleSort(pieces));
Insertion kind
Insertion kind is any other easy set of rules that builds the general taken care of array one merchandise at a time, and it’s named like this for the way in which smaller parts are inserted into their proper positions within the taken care of array.
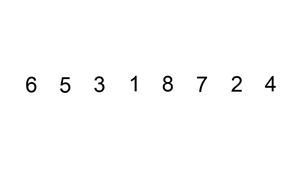
Historical past of Insertion kind
In The Artwork of Pc Programming, Knuth feedback that insertion kind “used to be discussed through John Mauchly as early as 1946, within the first revealed dialogue of laptop sorting”, describing it as a “herbal” set of rules that may simply be understood and carried out.
By means of the past due Fifties, Donald L. Shell made collection of enhancements in his shell kind manner (coated underneath), which compares parts separated through a distance that decreases on every cross, lowering the set of rules’s complexity to $O(n^{3/2})$
and $O(n^{4/3})$
in two other variants. This may no longer sound as a lot, however it’s reasonably an important growth for sensible packages!
Technical Notes: $O(n^{3/2})$
and $O(n^{4/3})$
complexities are extra environment friendly than $O(n^2)$
complexity, which means they take much less time to complete. It is because they don’t wish to carry out as many comparisons as $O(n^2)$
complexity.
For instance, it will take one 2d to kind an array of ten numbers the usage of a $O(n^2)$
set of rules, however it would take 0.5 seconds to kind the similar array the usage of a $O(n^{3/2})$
set of rules. It is because the set of rules can carry out fewer comparisons when the usage of the $O(n^{3/2})$
set of rules, leading to a quicker runtime.
In 2006 Bender, Martin Farach-Colton, and Mosteiro revealed a brand new variant of insertion kind known as library kind or “gapped insertion kind”, which leaves a small collection of unused areas (or “gaps”) unfold right through the array, additional making improvements to the working time to $O(n log n)$
.
Technical Notes: $O(n log n)$
complexity is extra environment friendly than $O(n^2)$
complexity, and $O(n^{3/2})$
and $O(n^{4/3})$
complexities. It is because it makes use of a divide-and-conquer means, which means that that it could wreck the issue into smaller items and remedy them extra temporarily.
For instance, it will take one 2d to kind an array of ten numbers the usage of a $O(n^2)$
set of rules, 0.5 seconds to kind the similar array the usage of a $O(n^{3/2})$
set of rules, however it would take 0.1 seconds to kind the similar array the usage of a $O(n log n)$
set of rules. It is because the set of rules can wreck the array into smaller items and remedy them in parallel, leading to a quicker runtime.
Benefits and downsides of insertion kind
Insertion kind is continuously utilized in follow for small information units or as a development block for extra complicated algorithms.
Similar to with bubble kind, its worst-case and average-case time complexity is $O(n^2)$
. However in contrast to bubble kind, insertion kind can be utilized to kind information units in-place, which means that it doesn’t require further reminiscence to retailer intermediate effects.
Use instances for insertion kind
Easy and environment friendly, insertion kind is continuously utilized in scenarios the place the enter information is already partly taken care of, or the place the scale of the enter information is somewhat small. It’s extensively utilized for sorting small information units and for development blocks for extra complicated algorithms, identical to bubble kind.
-
In part taken care of information. It’s well-suited for scenarios the place the knowledge is already partly taken care of. On this case, the set of rules can temporarily insert new parts into their proper positions with out the desire for complicated sorting operations.
-
On-line sorting. It’s continuously used for on-line sorting packages the place the enter information isn’t identified upfront. In those instances, the set of rules can incrementally kind the enter information because it’s gained.
-
Adaptive sorting. Insertion kind is a candidate for adaptive sorting as a result of it could profit from present order within the enter information. Because the enter information turns into extra ordered, the set of rules’s efficiency improves.
Insertion kind implementation
- Take an unsorted listing and make a selection the primary merchandise as a “pivot”.
- Iterate during the listing, placing the pivot into its proper position within the taken care of listing.
- Repeat the method with the following merchandise within the listing.
- Proceed till the listing is taken care of.
Insertion kind in Python
def insertion_sort(pieces):
for i in fluctuate(1, len(pieces)):
j = i
whilst j > 0 and pieces[j-1] > pieces[j]:
pieces[j-1], pieces[j] = pieces[j], pieces[j-1]
j -= 1
go back pieces
pieces = [6,20,8,19,56,23,87,41,49,53]
print(insertion_sort(pieces))
Insertion kind in JavaScript
serve as insertionSort(pieces) {
for (let i = 1; i < pieces.size; i++) {
let j = i;
whilst (j > 0 && pieces[j - 1] > pieces[j]) {
let temp = pieces[j];
pieces[j] = pieces[j - 1];
pieces[j - 1] = temp;
j--;
}
}
go back pieces;
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(insertionSort(pieces));
Quicksort
Quicksort is a well-liked divide-and-conquer sorting set of rules in line with the main of partitioning an array into two sub-arrays — one containing parts smaller than a “pivot” part and the opposite containing parts greater than the pivot part. The 2 sub-arrays are then taken care of recursively.
The fundamental steps of quicksort come with:
- Select a pivot part from the array.
- Partition the array into two sub-arrays, one containing parts smaller than the pivot and the opposite containing parts greater than the pivot.
- Type the 2 sub-arrays recursively the usage of quicksort.
- Mix the 2 taken care of sub-arrays.
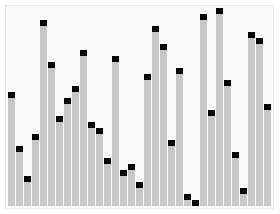
The historical past of quicksort
Quicksort used to be invented through Tony Hoare in 1959. Hoare used to be operating on the Elliott Brothers laptop corporate in Britain when he advanced the set of rules so that you could kind phrases within the reminiscence of the Ferranti Mark I laptop.
Quicksort used to be first of all revealed as a analysis paper in 1961, and it temporarily turned into one of the crucial extensively used sorting algorithms because of its simplicity, potency, and simplicity of implementation.
Benefits of quicksort
- It has a mean case time complexity of
$O(n log n)$
. - It calls for little or no further reminiscence, because it types the array in position.
- It’s simple to enforce and is extensively understood.
- It could possibly simply be parallelized.
Drawbacks of quicksort
Its worst-case time complexity is $O(n^2)$
when the pivot is selected poorly, making it much less environment friendly than different algorithms like merge kind or heapsort in positive scenarios.
Technical Observe: we don’t need to select a pivot that’s too small or too giant, or the set of rules will run in quadratic time. The perfect can be to select the median because the pivot, however it’s no longer all the time conceivable until we now have prior wisdom of the knowledge distribution.
Use instances of quicksort
As a extremely environment friendly sorting set of rules, quicksort has a variety of packages.
-
Massive information units. Its average-case time complexity is
$O(n log n)$
, which means that that it could kind massive quantities of information temporarily. -
Random information. It plays properly on randomly ordered information, as it is dependent upon the pivot part to divide the knowledge into two sub-arrays, that are then taken care of recursively. When the knowledge is random, the pivot part is perhaps on the subject of the median, which results in excellent efficiency.
-
Parallel processing. It could possibly simply be parallelized, which makes it very best for sorting massive information units on multi-core processors. By means of dividing the knowledge into smaller sub-arrays, the set of rules will also be carried out on a couple of cores concurrently, resulting in quicker efficiency.
-
Exterior sorting. It’s continuously used as a part of an exterior sorting set of rules, which is used to kind information that’s too massive to suit into reminiscence. On this case, the knowledge is taken care of into chunks, that are then merged the usage of a merge-sort set of rules.
-
Knowledge compression. It’s utilized in some information compression algorithms, such because the Burrows-Wheeler change into, which is used within the bzip2 compression instrument. The set of rules is used to kind the knowledge within the Burrows-Wheeler matrix, which is then remodeled to provide the compressed information.
Quicksort implementation
- Use a “pivot” level, preferably the median, to divide the listing into two portions.
- Briefly kind the left section and the suitable section.
- Proceed till the listing is taken care of.
Quicksort in Python
def quick_sort(pieces):
if len(pieces) > 1:
pivot = pieces[0]
left = [i for i in items[1:] if i < pivot]
proper = [i for i in items[1:] if i >= pivot]
go back quick_sort(left) + [pivot] + quick_sort(proper)
else:
go back pieces
pieces = [6,20,8,19,56,23,87,41,49,53]
print(quick_sort(pieces))
Quicksort in JavaScript
serve as quickSort(pieces) {
if (pieces.size > 1) {
let pivot = pieces[0];
let left = [];
let proper = [];
for (let i = 1; i < pieces.size; i++) {
if (pieces[i] < pivot) {
left.push(pieces[i]);
} else {
proper.push(pieces[i]);
}
}
go back quickSort(left).concat(pivot, quickSort(proper));
} else {
go back pieces;
}
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(quickSort(pieces));
Bucket kind
Bucket kind is an invaluable set of rules for sorting uniformly allotted information, and it could simply be parallelized for progressed efficiency.
The fundamental steps of bucket kind come with:
- Create an array of empty buckets.
- Scatter the enter information into the buckets in keeping with an outlined serve as.
- Type every bucket the usage of any other set of rules or recursively with bucket kind.
- Acquire the taken care of parts from every bucket into the unique array.
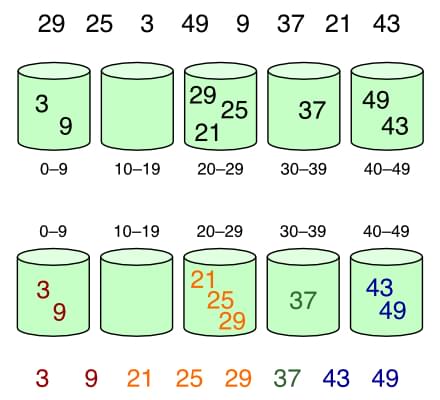
Benefits of bucket kind
- It’s environment friendly for uniformly allotted information, with an average-case time complexity of
$O(n+okay)$
, the place$n$
is the collection of parts and$okay$
is the collection of buckets. - It could possibly simply be parallelized, permitting it to profit from a couple of cores in fashionable processors.
- It’s strong, which means that it preserves the relative order of equivalent parts within the authentic array.
- It may be used for information with non-uniform distributions through adjusting the bucket serve as.
Technical Observe: $O(n+okay)$
complexity is extra environment friendly than $O(n^2)$
complexity, $O(n^{3/2})$
and $O(n^{4/3})$
complexities, and $O(n log n)$
complexity. It is because it handiest has to accomplish a linear collection of operations, without reference to the scale of the enter.
*For instance, believe an set of rules that types an array of numbers. It is going to take one 2d to kind an array of ten numbers the usage of a $O(n^2)$
set of rules, 0.5 seconds to kind the similar array the usage of a $O(n^{3/2})$
set of rules, 0.1 seconds to kind the similar array the usage of a $O(n log n)$
set of rules, however it would take 0.05 seconds to kind the similar array the usage of a $O(n+okay)$
set of rules. It is because the set of rules doesn’t wish to carry out as many comparisons.
Drawbacks of bucket kind
Bucket kind is much less environment friendly than different sorting algorithms on information that isn’t uniformly allotted, with a worst-case efficiency of $O(n^2)$
. Moreover, it calls for further reminiscence to retailer the buckets, which is usually a downside for extraordinarily massive information units.
The historical past of bucket kind
The are implementations of bucket kind already within the Fifties, with resources claiming the process has been round because the Forties.
Both method, it’s nonetheless in fashionable use at the present time.
Use instances for bucket kind
Similar to quicksort, bucket kind can simply be parallelized and used for exterior sorting, however bucket kind is especially helpful when coping with uniformly allotted information.
-
Sorting floating-point numbers. On this case, the variety is split into a hard and fast collection of buckets, every of which represents a sub-range of the enter information. The numbers are then positioned into their corresponding buckets and taken care of the usage of any other set of rules, corresponding to insertion kind. In the end, the taken care of information is concatenated right into a unmarried array.
-
Sorting strings. Strings are grouped into buckets in line with the primary letter of the string. The strings in every bucket are then taken care of the usage of any other set of rules, or recursively with bucket kind. This procedure is repeated for every next letter within the strings till all of the set is taken care of.
-
Histogram era. This can be utilized to generate histograms of information, that are used to constitute the frequency distribution of a collection of values. On this case, the variety of information is split into a hard and fast collection of buckets, and the collection of values in every bucket is counted. The ensuing histogram can be utilized to visualise the distribution of the knowledge.
Bucket kind implementation
- Cut up a listing of things into “buckets”.
- Every bucket is taken care of the usage of a distinct sorting set of rules.
- The buckets are then merged again into one taken care of listing.
Bucket kind in Python
def bucket_sort(pieces):
buckets = [[] for _ in fluctuate(len(pieces))]
for merchandise in pieces:
bucket = int(merchandise/len(pieces))
buckets[bucket].append(merchandise)
for bucket in buckets:
bucket.kind()
go back [item for bucket in buckets for item in bucket]
pieces = [6,20,8,19,56,23,87,41,49,53]
print(bucket_sort(pieces))
Bucket kind in JavaScript
serve as bucketSort(pieces) {
let buckets = new Array(pieces.size);
for (let i = 0; i < buckets.size; i++) {
buckets[i] = [];
}
for (let j = 0; j < pieces.size; j++) {
let bucket = Math.flooring(pieces[j] / pieces.size);
buckets[bucket].push(pieces[j]);
}
for (let okay = 0; okay < buckets.size; okay++) {
buckets[k].kind();
}
go back [].concat(...buckets);
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(bucketSort(pieces));
Shell kind
Shell kind makes use of an insertion kind set of rules, however as an alternative of sorting all of the listing without delay, the listing is split into smaller sub-lists. Those sub-lists are then taken care of the usage of an insertion kind set of rules, thus lowering the collection of exchanges had to kind the listing.
Sometimes called “Shell’s manner”, it really works through first defining a series of integers known as the increment series. The increment series is used to resolve the scale of the sub-lists that might be taken care of independently. Probably the most recurrently used increment series is “the Knuth series”, which is outlined as follows (the place $h$
is period with preliminary worth and $n$ is the size of the listing):
h = 1
whilst h < n:
h = 3*h + 1
As soon as the increment series has been outlined, the Shell kind set of rules proceeds through sorting the sub-lists of parts. The sub-lists are taken care of the usage of an insertion kind set of rules, with the increment series because the step dimension. The set of rules types the sub-lists, beginning with the most important increment after which iterating all the way down to the smallest increment.
The set of rules stops when the increment dimension is 1
, at which level it’s identical to an ordinary insertion kind set of rules.
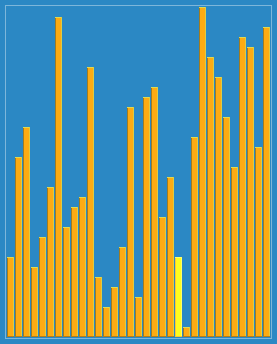
The historical past of shell kind
Shell kind used to be invented through Donald Shell in 1959 as a variation of insertion kind, which targets to enhance its efficiency through breaking the unique listing into smaller sub-lists and sorting those sub-lists independently.
Benefits of shell kind
- It’s a generalization of insertion kind and subsequently simple to grasp and enforce.
- It has a time complexity that’s higher than
$O(n^2)$
for plenty of sequences of enter information. - It’s an in-place sorting set of rules, which means that it doesn’t require further reminiscence.
Drawbacks of shell kind
It may be tricky to expect the time complexity of shell sorting, because it is dependent upon the number of increment series.
Use sases for shell kind
Shell kind is a general-purpose set of rules for sorting information in numerous packages, specifically when sorting massive information units like with quicksort and bucket kind.
-
Sorting most commonly taken care of information. Shell kind reduces the collection of comparisons and swaps required to kind information. This makes it quicker than different sorting algorithms corresponding to quicksort or merge kind on this explicit situation.
-
Sorting arrays with a small collection of inversions. Inversion is a measure of ways unsorted an array is, and is outlined because the collection of pairs of parts which can be within the flawed order. Shell kind is extra environment friendly than another algorithms corresponding to bubble kind or insertion kind when sorting arrays with a small collection of inversions.
-
In-place sorting. Shell kind doesn’t require further reminiscence to kind the enter, making it a contender for in-place sorting. This makes it helpful in scenarios the place reminiscence is proscribed or when further reminiscence utilization is unwanted.
-
Sorting in a allotted surroundings. By means of dividing the enter information into smaller sub-lists and sorting them independently, every sub-list will also be taken care of on a separate processor or node, lowering the time required to kind the knowledge.
Shell kind implementation
- Divide a listing of things into “buckets” in line with some standards
- Type every bucket personally
- Mix the taken care of buckets
Shell kind implementation in Python
def shell_sort(pieces):
sublistcount = len(pieces)//2
whilst sublistcount > 0:
for get started in fluctuate(sublistcount):
gap_insertion_sort(pieces, get started, sublistcount)
sublistcount = sublistcount // 2
go back pieces
def gap_insertion_sort(pieces, get started, hole):
for i in fluctuate(get started+hole, len(pieces), hole):
currentvalue = pieces[i]
place = i
whilst place >= hole and pieces[position-gap] > currentvalue:
pieces[position] = pieces[position-gap]
place = place-hole
pieces[position] = currentvalue
pieces = [6,20,8,19,56,23,87,41,49,53]
print(shell_sort(pieces))
Shell kind implementation in JavaScript
serve as shellSort(pieces) {
let sublistcount = Math.flooring(pieces.size / 2);
whilst (sublistcount > 0) {
for (let get started = 0; get started < sublistcount; get started++) {
gapInsertionSort(pieces, get started, sublistcount);
}
sublistcount = Math.flooring(sublistcount / 2);
}
go back pieces;
}
serve as gapInsertionSort(pieces, get started, hole) {
for (let i = get started + hole; i < pieces.size; i += hole) {
let currentValue = pieces[i];
let place = i;
whilst (place >= hole && pieces[position - gap] > currentValue) {
pieces[position] = pieces[position - gap];
place = place - hole;
}
pieces[position] = currentValue;
}
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(shellSort(pieces));
Merge kind
The fundamental thought of merge kind is to divide the enter listing in part, kind every part recursively the usage of merge kind, after which merge the 2 taken care of halves again in combination. The merge step is carried out through many times evaluating the primary part of every part and including the smaller of the 2 to the taken care of listing. This procedure is repeated till all parts had been merged again in combination.
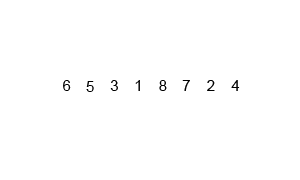
Benefits of merge kind
Merge kind has a time complexity of $O(n log n)$
within the worst-case situation, which makes it extra environment friendly than different fashionable sorting algorithms corresponding to bubble kind, insertion kind, or variety kind.
Merge kind could also be a set of rules, which means that it preserves the relative order of equivalent parts.
Drawbacks of merge kind
Merge kind has some disadvantages in the case of reminiscence utilization. The set of rules calls for further reminiscence to retailer the 2 halves of the listing all over the divide step, in addition to further reminiscence to retailer the general taken care of listing all over the merge step. It is a worry when sorting very massive lists.
The historical past of merge kind
Merge kind used to be invented through John von Neumann in 1945, as a comparison-based sorting set of rules that works through dividing an enter listing into smaller sub-lists, sorting the ones sub-lists recursively, after which merging them again in combination to provide the general taken care of listing.
Use instances for merge kind
Merge kind is a general-purpose sorting set of rules that may be parallelized to kind massive information units and in exterior sorting (à los angeles quicksort and bucket kind), and it’s additionally recurrently use as a development block for extra complicated algorithms (like bubble kind and insertion kind).
-
Solid sorting. strong sorting for merge kind implies that it preserves the relative order of equivalent parts. This makes it helpful in scenarios the place keeping up the order of equivalent parts is vital, corresponding to in monetary packages or when sorting information for visualisation functions.
-
Imposing binary seek. It’s used to successfully seek for a particular part in a taken care of listing, because it is dependent upon a taken care of enter. Merge kind can be utilized to successfully kind the enter for binary seek and different an identical algorithms.
Merge kind implementation
- Use recursion to separate a listing into smaller, taken care of sub-lists
- Merge the sub-lists again in combination, evaluating and sorting pieces as they’re merged
Merge kind implementation in Python
def merge_sort(pieces):
if len(pieces) <= 1:
go back pieces
mid = len(pieces) // 2
left = pieces[:mid]
proper = pieces[mid:]
left = merge_sort(left)
proper = merge_sort(proper)
go back merge(left, proper)
def merge(left, proper):
merged = []
left_index = 0
right_index = 0
whilst left_index < len(left) and right_index < len(proper):
if left[left_index] > proper[right_index]:
merged.append(proper[right_index])
right_index += 1
else:
merged.append(left[left_index])
left_index += 1
merged += left[left_index:]
merged += proper[right_index:]
go back merged
pieces = [6,20,8,19,56,23,87,41,49,53]
print(merge_sort(pieces))
Merge kind implementation in JavaScript
serve as mergeSort(pieces) {
if (pieces.size <= 1) {
go back pieces;
}
let mid = Math.flooring(pieces.size / 2);
let left = pieces.slice(0, mid);
let proper = pieces.slice(mid);
go back merge(mergeSort(left), mergeSort(proper));
}
serve as merge(left, proper) {
let merged = [];
let leftIndex = 0;
let rightIndex = 0;
whilst (leftIndex < left.size && rightIndex < proper.size) {
if (left[leftIndex] > proper[rightIndex]) {
merged.push(proper[rightIndex]);
rightIndex++;
} else {
merged.push(left[leftIndex]);
leftIndex++;
}
}
go back merged.concat(left.slice(leftIndex)).concat(proper.slice(rightIndex));
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(mergeSort(pieces));
Variety kind
Variety kind many times selects the smallest part from an unsorted portion of a listing and swaps it with the primary part of the unsorted portion. This procedure continues till all of the listing is taken care of.
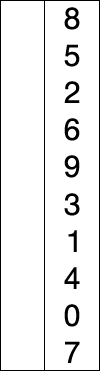
The historical past of variety kind
Variety kind is a straightforward and intuitive sorting set of rules that’s been round because the early days of laptop science. It’s most likely that an identical algorithms have been advanced independently through researchers within the Fifties.
It used to be one of the crucial first sorting algorithms to be advanced, and it stays a well-liked set of rules for tutorial functions and for easy sorting duties.
Benefits of variety kind
Variety kind is utilized in some packages the place simplicity and simplicity of implementation are extra vital than potency. It’s additionally helpful as a instructing software for introducing scholars to sorting algorithms and their houses, because it’s simple to grasp and enforce.
Drawbacks of variety kind
In spite of its simplicity, variety kind isn’t very environment friendly in comparison to different sorting algorithms corresponding to merge kind or quicksort. It has a worst-case time complexity of $O(n^2)$
, and it could take a very long time to kind massive lists.
Variety kind additionally isn’t a strong sorting set of rules, which means that it won’t keep the order of equivalent parts.
Use instances for variety kind
Variety kind is very similar to bubble kind and insertion kind in that during can be utilized to kind small information units, and its simplicity additionally makes it a useful gizmo for instructing and studying about sorting algorithms. Different makes use of come with:
-
Sorting information with restricted reminiscence. It calls for just a consistent quantity of extra reminiscence to accomplish the type, making it helpful in scenarios the place reminiscence utilization is proscribed.
-
Sorting information with distinctive values. It doesn’t rely at the enter being most commonly taken care of, making it a good selection for information units with distinctive values the place different sorting algorithms will have to accomplish further assessments or optimizations.
Variety kind implementation
- Iterate during the listing, settling on the bottom merchandise
- Change the bottom merchandise with the thing on the present place
- Repeat the method for the remainder of the listing
Variety kind implementation in Python
def selection_sort(pieces):
for i in fluctuate(len(pieces)):
min_idx = i
for j in fluctuate(i+1, len(pieces)):
if pieces[min_idx] > pieces[j]:
min_idx = j
pieces[i], pieces[min_idx] = pieces[min_idx], pieces[i]
go back pieces
pieces = [6,20,8,19,56,23,87,41,49,53]
print(selection_sort(pieces))
Variety kind implementation in JavaScript
serve as selectionSort(pieces) {
let minIdx;
for (let i = 0; i < pieces.size; i++) {
minIdx = i;
for (let j = i + 1; j < pieces.size; j++) {
if (pieces[j] < pieces[minIdx]) {
minIdx = j;
}
}
let temp = pieces[i];
pieces[i] = pieces[minIdx];
pieces[minIdx] = temp;
}
go back pieces;
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(selectionSort(pieces));
Radix kind
The fundamental thought at the back of radix kind is to kind information through grouping it through every digit within the numbers or characters being taken care of, from proper to left or left to proper. This procedure is repeated for every digit, leading to a taken care of listing.
Its worst-case efficiency is ${O(wcdot n)}$
, the place $n$
is the collection of keys, and $w$
is the important thing size.
The historical past of radix kind
Radix kind used to be first presented through Herman Hollerith within the past due nineteenth century so that you could successfully kind information on punched playing cards, the place every column represented a digit within the information.
It used to be later tailored and popularized through a number of researchers within the mid-Twentieth century to kind binary information through grouping the knowledge through every bit within the binary illustration. However it’s extensively utilized to kind string information, the place every personality is handled as a digit within the kind.
Lately, radix kind has noticed renewed hobby as a sorting set of rules for parallel and allotted computing environments, because it’s simply parallelizable and can be utilized to kind massive information units in a allotted style.
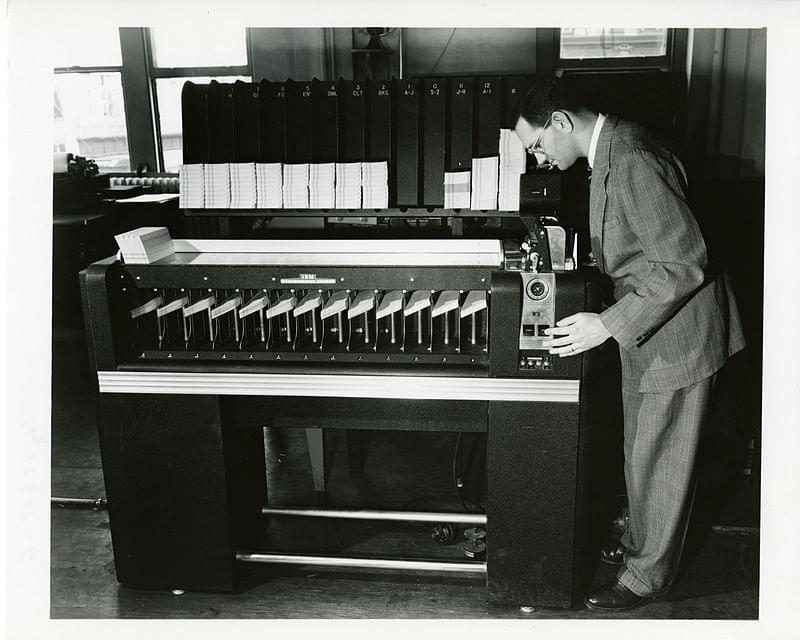
Benefits of radix kind
Radix kind is a linear-time sorting set of rules, which means that its time complexity is proportional to the scale of the enter information. This makes it an effective set of rules for sorting massive information units, even if it is probably not as environment friendly as different sorting algorithms for smaller information units.
Its linear-time complexity and steadiness make it a useful gizmo for sorting massive information units, and its parallelizability (yeah, that’s a real phrase) makes it helpful for sorting information in allotted computing environments.
Radix kind could also be a strong sorting set of rules, which means that it preserves the relative order of equivalent parts.
Use instances for radix kind
Radix kind can be utilized in more than a few packages the place environment friendly sorting of huge information units is needed. It’s specifically helpful for sorting string information and fixed-length keys, and can be utilized in parallel and allotted computing environments.
-
Parallel processing. Radix kind is continuously most well-liked for sorting massive information units (over merge kind, quicksort and bucket kind). And prefer bucket kind, radix can kind string information successfully, which makes it appropriate for herbal language processing packages.
-
Sorting information with fixed-length keys. Radix kind is especially environment friendly when sorting information with fixed-length keys, as it could carry out the type through analyzing every key one digit at a time.
Radix kind implementation
- Examine the digits of every merchandise within the listing.
- Team the pieces in keeping with the digits.
- Type the teams through dimension.
- Recursively kind every team till every merchandise is in its proper place.
Radix kind implementation in Python
def radix_sort(pieces):
max_length = False
tmp, placement = -1, 1
whilst no longer max_length:
max_length = True
buckets = [list() for _ in range(10)]
for i in pieces:
tmp = i // placement
buckets[tmp % 10].append(i)
if max_length and tmp > 0:
max_length = False
a = 0
for b in fluctuate(10):
dollar = buckets[b]
for i in dollar:
pieces[a] = i
a += 1
placement *= 10
go back pieces
pieces = [6,20,8,19,56,23,87,41,49,53]
print(radix_sort(pieces))
Radix kind implementation in JavaScript
serve as radixSort(pieces) {
let maxLength = false;
let tmp = -1;
let placement = 1;
whilst (!maxLength) {
maxLength = true;
let buckets = Array.from({ size: 10 }, () => []);
for (let i = 0; i < pieces.size; i++) {
tmp = Math.flooring(pieces[i] / placement);
buckets[tmp % 10].push(pieces[i]);
if (maxLength && tmp > 0) {
maxLength = false;
}
}
let a = 0;
for (let b = 0; b < 10; b++) {
let dollar = buckets[b];
for (let j = 0; j < dollar.size; j++) {
pieces[a] = dollar[j];
a++;
}
}
placement *= 10;
}
go back pieces;
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(radixSort(pieces));
Comb kind
Comb kind compares pairs of parts which can be a undeniable distance aside, and change them in the event that they’re out of order. The space between the pairs is first of all set to the scale of the listing being taken care of, and is then diminished through an element (known as the “shrink issue”) with every cross, till it reaches a minimal worth of $1$
. This procedure is repeated till the listing is totally taken care of.
The brush kind set of rules is very similar to the bubble kind set of rules, however with a bigger hole between the when put next parts. This greater hole permits for greater values to transport extra temporarily to their proper place within the listing.
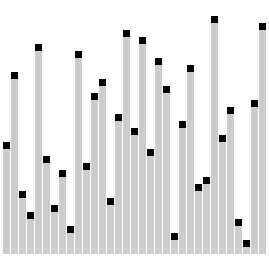
okay = 1.24733
. Symbol supply: Wikimedia Commons, CC BY-SA 3.0.The historical past of comb kind
The brush kind set of rules is a somewhat contemporary sorting set of rules that used to be first presented in 1980 through Włodzimierz Dobosiewicz and Artur Borowy. The set of rules used to be impressed through the speculation of the usage of a comb to straighten out tangled hair, and it makes use of a an identical procedure to straighten out a listing of unsorted values.
Benefits of comb kind
Comb kind has a worst-case time complexity of $O(n^2)$
, however in follow it’s continuously quicker than different $O(n^2)$
sorting algorithms corresponding to bubble kind, because of its use of the shrink issue. The shrink issue permits the set of rules to temporarily transfer massive values in opposition to their proper place, lowering the collection of passes required to completely kind the listing.
Use instances for comb kind
Comb kind is a somewhat easy and environment friendly sorting set of rules that has a number of use instances in more than a few packages.
-
Sorting information with a wide variety of values. The usage of a bigger hole between when put next parts permits greater values to transport extra temporarily to their proper place within the listing.
-
Sorting information in real-time packages. As a strong sorting set of rules, comb kind preserves the relative order of equivalent parts. This makes it helpful for sorting information in real-time packages the place the order of equivalent parts must be preserved.
-
Sorting information in memory-constrained environments. Comb kind doesn’t require further reminiscence to kind the knowledge. This makes it helpful for sorting information in memory-constrained environments the place further reminiscence isn’t to be had.
Comb kind implementation
- Get started with a big hole between pieces.
- Examine pieces on the ends of the distance and change them if they’re within the flawed order.
- Scale back the distance and repeat the method till the distance is
1
. - End with a bubble kind at the final pieces.
Comb kind implementation in Python
def comb_sort(pieces):
hole = len(pieces)
shrink = 1.3
taken care of = False
whilst no longer taken care of:
hole //= shrink
if hole <= 1:
taken care of = True
else:
for i in fluctuate(len(pieces)-hole):
if pieces[i] > pieces[i+gap]:
pieces[i],pieces[i+gap] = pieces[i+gap],pieces[i]
go back bubble_sort(pieces)
def bubble_sort(pieces):
for i in fluctuate(len(pieces)):
for j in fluctuate(len(pieces)-1-i):
if pieces[j] > pieces[j+1]:
pieces[j], pieces[j+1] = pieces[j+1], pieces[j]
go back pieces
pieces = [6,20,8,19,56,23,87,41,49,53]
print(comb_sort(pieces))
Comb kind implementation in JavaScript
serve as combSort(pieces) {
let hole = pieces.size;
let shrink = 1.3;
let taken care of = false;
whilst (!taken care of) {
hole = Math.flooring(hole / shrink);
if (hole <= 1) {
taken care of = true;
} else {
for (let i = 0; i < pieces.size - hole; i++) {
if (pieces[i] > pieces[i + gap]) {
let temp = pieces[i];
pieces[i] = pieces[i + gap];
pieces[i + gap] = temp;
}
}
}
}
go back bubbleSort(pieces);
}
serve as bubbleSort(pieces) {
let swapped;
do {
swapped = false;
for (let i = 0; i < pieces.size - 1; i++) {
if (pieces[i] > pieces[i + 1]) {
let temp = pieces[i];
pieces[i] = pieces[i + 1];
pieces[i + 1] = temp;
swapped = true;
}
}
} whilst (swapped);
go back pieces;
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(combSort(pieces));
Timsort
The Timsort set of rules works through dividing the enter information into smaller sub-arrays, after which the usage of insertion kind to kind those sub-arrays. Those taken care of sub-arrays are then mixed the usage of merge kind to provide an absolutely taken care of array.
Timsort has a worst-case time complexity of $O(n log n)$
, which makes it environment friendly for sorting massive information units. It’s additionally a strong sorting set of rules, which means that it preserves the relative order of equivalent parts.
Benefits of Timsort
One of the most key options of Timsort is its talent to care for various kinds of information successfully. It does this through detecting “runs”, that are sequences of parts which can be already taken care of. Timsort then combines those runs in some way that minimizes the collection of comparisons and swaps required to provide an absolutely taken care of array.
Any other vital characteristic of Timsort is its talent to care for information that’s partly taken care of. On this case, Timsort can locate the partly taken care of areas and use insertion kind to temporarily kind them, lowering the time required to completely kind the knowledge.
The historical past of Timsort
Timsort used to be advanced through Tim Peters in 2002 to be used within the Python programming language. It’s a hybrid sorting set of rules that makes use of a mix of insertion kind and merge kind ways, and is designed to successfully kind numerous various kinds of information.
It’s since been followed through a number of different programming languages, together with Java and C#, because of its potency and flexibility in dealing with various kinds of information.
Use instances for Timsort
As a complicated set of rules, Timsort can be utilized when sorting information on memory-constrained methods.
-
Sorting in programming languages. Timsort is continuously used because the default sorting set of rules in those languages on account of its potency and talent to care for various kinds of information.
-
Sorting real-world information. Timsort is especially environment friendly when sorting real-world information that can be partly taken care of or include already taken care of sub-arrays, because it’s ready to locate those runs and use insertion kind to temporarily kind them, lowering the time required to completely kind the knowledge.
-
Sorting information with differing types. It’s designed to successfully care for various kinds of information, together with numbers, strings, and customized gadgets. It could possibly locate runs of information with the similar sort and mix them successfully the usage of merge kind, lowering the collection of comparisons and swaps required.
Timsort implementation
- Take an unsorted listing and breaks it into smaller, taken care of sub-lists.
- Merge the sub-lists to shape a bigger, taken care of listing.
- Repeat the method till all of the listing is taken care of.
Timsort implementation in Python
def insertion_sort(arr, left=0, proper=None):
if proper is None:
proper = len(arr) - 1
for i in fluctuate(left + 1, proper + 1):
key_item = arr[i]
j = i - 1
whilst j >= left and arr[j] > key_item:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key_item
go back arr
def merge(left, proper):
if no longer left:
go back proper
if no longer proper:
go back left
if left[0] < proper[0]:
go back [left[0]] + merge(left[1:], proper)
go back [right[0]] + merge(left, proper[1:])
def timsort(arr):
min_run = 32
n = len(arr)
for i in fluctuate(0, n, min_run):
insertion_sort(arr, i, min((i + min_run - 1), n - 1))
dimension = min_run
whilst dimension < n:
for get started in fluctuate(0, n, dimension * 2):
midpoint = get started + dimension - 1
finish = min((get started + dimension * 2 - 1), (n-1))
merged_array = merge(
left=arr[start:midpoint + 1],
proper=arr[midpoint + 1:end + 1]
)
arr[start:start + len(merged_array)] = merged_array
dimension *= 2
go back arr
pieces = [6,20,8,19,56,23,87,41,49,53]
print(timsort(pieces))
Timsort implementation in JavaScript
serve as insertionSort(arr, left = 0, proper = arr.size - 1) {
for (let i = left + 1; i <= proper; i++) {
const keyItem = arr[i];
let j = i - 1;
whilst (j >= left && arr[j] > keyItem) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = keyItem;
}
go back arr;
}
serve as merge(left, proper) {
let i = 0;
let j = 0;
const merged = [];
whilst (i < left.size && j < proper.size) {
if (left[i] < proper[j]) {
merged.push(left[i]);
i++;
} else {
merged.push(proper[j]);
j++;
}
}
go back merged.concat(left.slice(i)).concat(proper.slice(j));
}
serve as timsort(arr) {
const minRun = 32;
const n = arr.size;
for (let i = 0; i < n; i += minRun) {
insertionSort(arr, i, Math.min(i + minRun - 1, n - 1));
}
let dimension = minRun;
whilst (dimension < n) {
for (let get started = 0; get started < n; get started += dimension * 2) {
const midpoint = get started + dimension - 1;
const finish = Math.min(get started + dimension * 2 - 1, n - 1);
const merged = merge(
arr.slice(get started, midpoint + 1),
arr.slice(midpoint + 1, finish + 1)
);
arr.splice(get started, merged.size, ...merged);
}
dimension *= 2;
}
go back arr;
}
let pieces = [6, 20, 8, 19, 56, 23, 87, 41, 49, 53];
console.log(timsort(pieces));
All Sorting Algorithms When put next
Observe that the time complexity and house complexity indexed within the desk are worst-case complexities, and precise efficiency would possibly range relying at the explicit implementation and enter information.
What’s the Maximum Commonplace Sorting Set of rules?
Probably the most recurrently used sorting set of rules is more than likely quicksort. It’s extensively utilized in many programming languages, together with C, C++, Java, and Python, in addition to in lots of instrument packages and libraries. Quicksort is liked for its potency and flexibility in dealing with various kinds of information, and is continuously used because the default sorting set of rules in programming languages and instrument frameworks.
Then again, different sorting algorithms like merge kind and Timsort also are recurrently utilized in more than a few packages because of their potency and distinctive options.
Ultimate Ideas
Figuring out the fundamentals of sorting algorithms is very important for someone eager about programming, information research, or laptop science. By means of figuring out the other sorting algorithms and their traits, you’ll enhance your talent to choose and enforce the most efficient set of rules in your explicit use case.
Your only option of sorting set of rules is dependent upon a number of components, together with the scale of the enter information, the distribution of the knowledge, the to be had reminiscence, and the specified time complexity.
Sorting algorithms will also be categorised in line with their time complexity, house complexity, in-place sorting, strong sorting, and adaptive sorting. It’s vital to grasp the traits and trade-offs of various sorting algorithms to choose probably the most suitable set of rules for a particular use case.
[ad_2]